Python for Machine Learning: A Data Scientist’s Step-by-Step Starter Guide
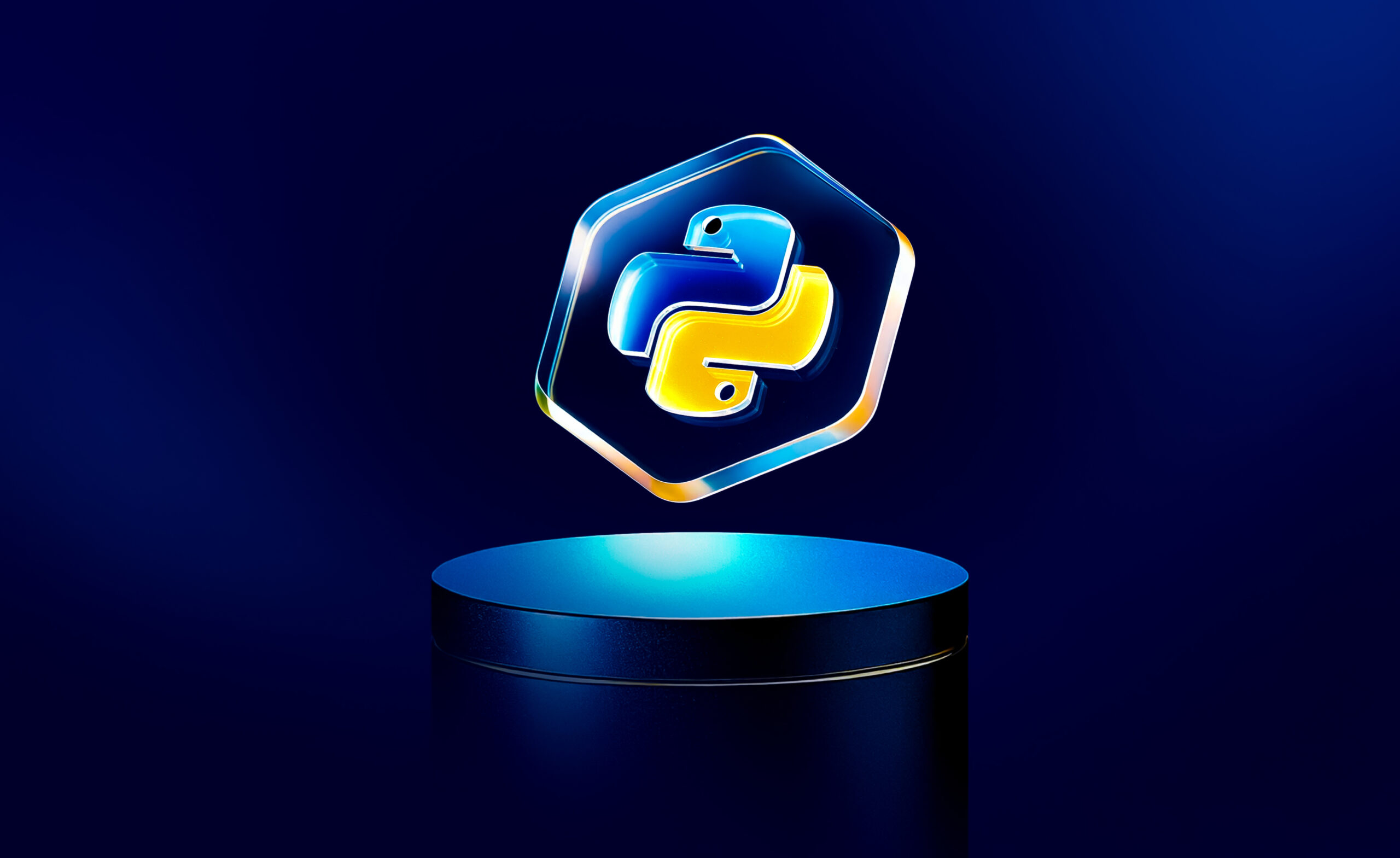
If you're diving into machine learning (ML), Python is your best friend. As someone who’s built everything from spam filters to recommendation engines, I’ll walk you through exactly how to get started—with the right tools, libraries, and best practices I wish I knew earlier.
1. Why Python for Machine Learning?
✅ Rich Ecosystem: Libraries like scikit-learn
, TensorFlow
, and PyTorch
make ML accessible.
✅ Easy to Learn: Clean syntax compared to languages like C++ or Java.
✅ Community Support: Tons of tutorials, Stack Overflow answers, and pre-trained models.
✅ Integration: Works seamlessly with data tools (Pandas, SQL, Spark).
🔹 Example: Companies like Netflix, Google, and Uber use Python for ML.
2. Setting Up Your Python ML Environment
Option 1: Local Setup (Recommended)
- Install Python 3.9+ (avoid Python 2!):
- Use a virtual environment (keeps dependencies clean):bashCopypython -m venv ml_env source ml_env/bin/activate # Linux/Mac ml_env\Scripts\activate # Windows
- Install key libraries:bashCopypip install numpy pandas scikit-learn matplotlib jupyter
Option 2: Cloud Notebooks (Quick Start)
- Google Colab (Free GPU!): colab.research.google.com
- Kaggle Notebooks: Great for practice datasets.
3. Essential Python Libraries for ML
Library | Purpose | Example Use Case |
---|---|---|
NumPy | Numerical computing | Matrix operations for ML models |
Pandas | Data manipulation | Cleaning CSV data before training |
Matplotlib | Visualization | Plotting model accuracy over time |
scikit-learn | Classic ML algorithms | Training a decision tree classifier |
TensorFlow/PyTorch | Deep learning | Building neural networks |
🔹 Example:
import pandas as pd from sklearn.linear_model import LogisticRegression # Load data data = pd.read_csv("titanic.csv") X = data[["Age", "Fare"]] y = data["Survived"] # Train model model = LogisticRegression() model.fit(X, y)
4. Your First ML Project: Step-by-Step
Step 1: Pick a Dataset
Start with small, clean datasets:
- Iris Dataset (Classification)
- Boston Housing (Regression)
Step 2: Preprocess Data
- Handle missing values:pythonCopydf[“Age”].fillna(df[“Age”].median(), inplace=True)
- Encode categorical variables:pythonCopyfrom sklearn.preprocessing import LabelEncoder df[“Gender”] = LabelEncoder().fit_transform(df[“Gender”])
Step 3: Train a Model
from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier # Split data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2) # Train model = RandomForestClassifier() model.fit(X_train, y_train) # Evaluate print("Accuracy:", model.score(X_test, y_test))
Step 4: Improve Your Model
- Hyperparameter Tuning: Use
GridSearchCV
:pythonCopyfrom sklearn.model_selection import GridSearchCV params = {“n_estimators”: [50, 100, 200]} grid = GridSearchCV(model, params, cv=5) grid.fit(X_train, y_train) - Feature Engineering: Add new features (e.g., “Family Size” = SibSp + Parch).
5. Avoiding Common Beginner Mistakes
❌ Using the wrong evaluation metric (e.g., accuracy for imbalanced datasets → use F1-score instead).
❌ Not splitting data into train/test sets (leading to overfitting).
❌ Ignoring feature scaling (critical for SVM, KNN).
🔹 Fix: Always:
- Start with simple models (linear regression before neural nets).
- Validate with cross-validation (
sklearn.model_selection.cross_val_score
).
6. Where to Go Next
Level Up Your Skills
📚 Books:
- “Hands-On Machine Learning with Scikit-Learn & TensorFlow” (Aurélien Géron)
- “Python for Data Analysis” (Wes McKinney)
🎓 Courses:
- Coursera: Andrew Ng’s ML Course (Theory)
- Kaggle Learn (Practical)
Practice Projects
- Predict Titanic Survival (Beginner)
- Build a Spam Classifier (Intermediate)
- Train a CNN for MNIST Digits (Advanced)
Final Thoughts
Python makes ML approachable, but real-world data is messy. The key is to:
- Start small (Iris dataset → custom projects).
- Learn the fundamentals (metrics, preprocessing).
- Build a portfolio (GitHub, Kaggle notebooks).
What’s your first ML project? Share in the comments!