ETL in Data Analysis: A Step-by-Step Guide to Extracting, Transforming, and Loading Data
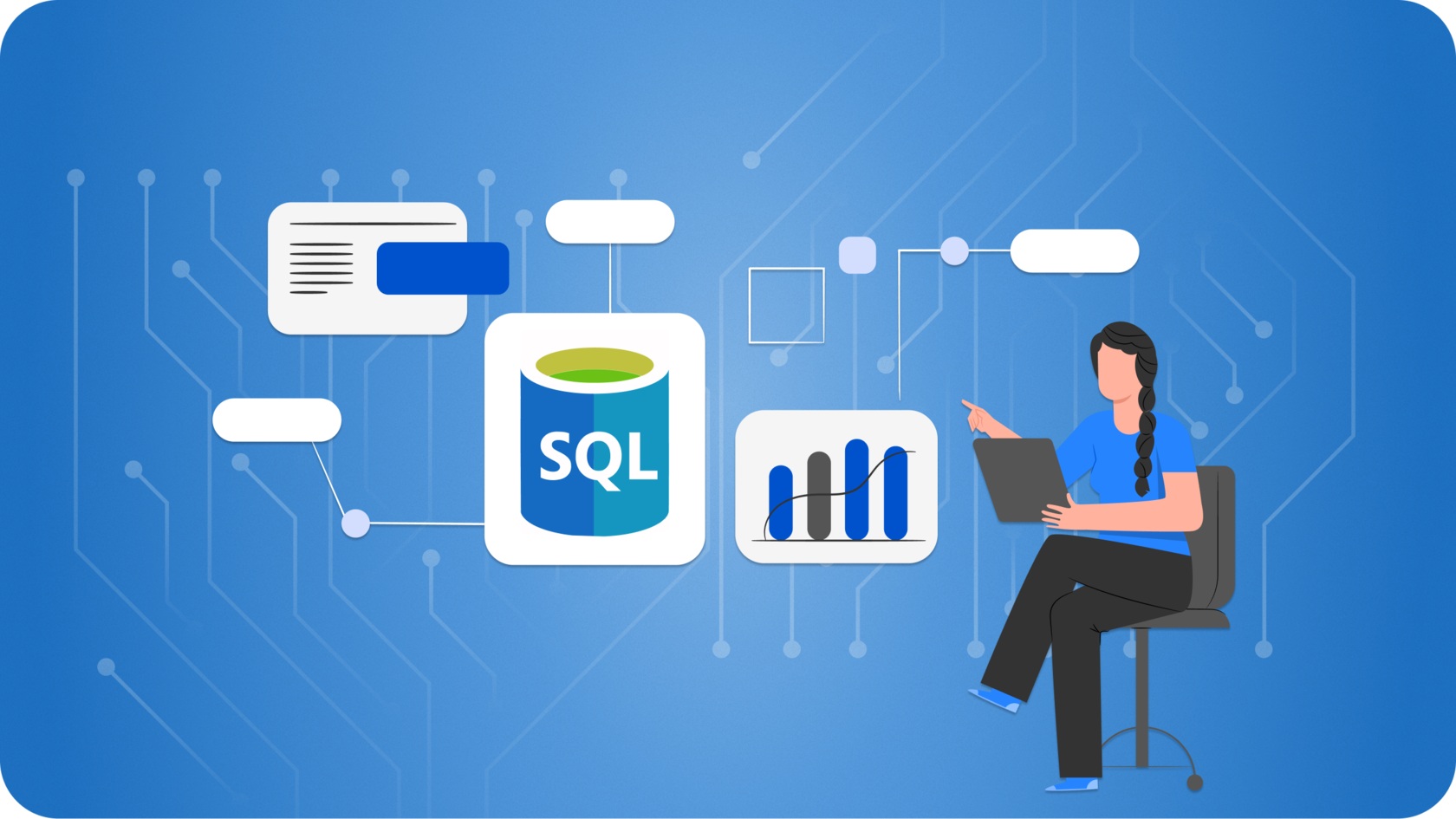
As a data analyst, I once spent three weeks manually cleaning a client’s sales data—until I discovered ETL (Extract, Transform, Load). Automating this process saved me 20+ hours per project and reduced errors by 90%.
In this guide, I’ll explain:
✔ What ETL is (and why it’s critical for data analysis).
✔ The 3 key stages (with real-world examples).
✔ Tools to automate ETL (from Excel to Python).
What is ETL?
ETL is the process of:
- Extracting data from sources (databases, APIs, files).
- Transforming it (cleaning, filtering, enriching).
- Loading it into a destination (database, dashboard).
Why it matters:
- Ensures consistent, analysis-ready data.
- Eliminates manual copy-pasting.
- Enables real-time analytics.
The 3 Stages of ETL
1. Extract: Pull Data from Sources
Sources:
- Databases (SQL, MongoDB).
- Cloud apps (Google Sheets, Salesforce).
- APIs (Twitter, weather data).
- Files (CSV, Excel, PDFs).
Example (Python):
import pandas as pd # Extract from CSV sales_data = pd.read_csv("sales_2023.csv") # Extract from API import requests weather_data = requests.get("https://api.weather.com/v1/data").json()
Common Issues:
- API rate limits.
- Inconsistent file formats.
2. Transform: Clean & Prepare Data
Key Tasks:
Task | Example | Tool |
---|---|---|
Cleaning | Fix missing values, typos. | Pandas (dropna() ) |
Filtering | Remove test entries. | SQL (WHERE clause) |
Enriching | Add weather data to sales records. | Python (merge() ) |
Aggregating | Sum daily sales by region. | Power Query (Group By) |
Example (Python):
# Clean: Fill missing values sales_data['region'].fillna('Unknown', inplace=True) # Enrich: Merge with customer data merged_data = pd.merge(sales_data, customers, on='customer_id') # Aggregate: Total sales per region sales_by_region = sales_data.groupby('region')['sales'].sum()
Watch Out For:
- Data type mismatches (e.g., dates as strings).
- Duplicate records.
3. Load: Send Data to Destination
Destinations:
- Databases (PostgreSQL, BigQuery).
- Data warehouses (Snowflake, Redshift).
- Dashboards (Power BI, Tableau).
Example (Python → PostgreSQL):
from sqlalchemy import create_engine engine = create_engine("postgresql://user:pass@localhost:5432/db") sales_by_region.to_sql('sales_report', engine, if_exists='replace')
Best Practices:
- Use upserts (update existing records).
- Log errors (e.g., failed API calls).
ETL Tools Comparison
Tool | Best For | Learning Curve |
---|---|---|
Excel Power Query | Small datasets, no coding. | Low |
Python (Pandas) | Custom pipelines. | Medium |
Apache Airflow | Scheduling complex ETL. | High |
Talend | Enterprise-grade ETL. | Medium |
Real-World ETL Example: Retail Sales
- Extract: Pull data from 10 store POS systems (CSV + API).
- Transform:
- Clean product names (e.g., “T-shirt” → “T-Shirt”).
- Flag outliers (sales > $10K manually verified).
- Load: Push to Power BI for daily dashboards.
Result: Reduced report generation time from 8 hours to 20 minutes.
ETL Mistakes to Avoid
- No backups (Always keep raw data).
- Hardcoding credentials (Use environment variables).
- Ignoring latency (Stream real-time data if needed).
Free Resources
Need help designing an ETL pipeline? DM me your data sources!
P.S. What’s your biggest ETL headache? Messy sources? Slow transforms? Let’s discuss! 💬
References: